WebAssembly is a low level code (similar to assembly), which could be written in C/C++ or Rust, compiled (to .wasm file), and run in a browser together with JavaScript.
To create WebAssembly binaries we will use Emscripten compiler.
Installation instructions could be found here. (Note you have to have Python installed on your computer).
In a nutshell it’s very simple:
# Get the emsdk repo
git clone https://github.com/emscripten-core/emsdk.git
# Enter that directory
cd emsdk
# Fetch the latest version of the emsdk (not needed the first time you clone)
git pull
# Download and install the latest SDK tools.
./emsdk install latest
# Make the "latest" SDK "active" for the current user. (writes .emscripten file)
./emsdk activate latest
# Activate PATH and other environment variables in the current terminal:
# on Linux
source ./emsdk_env.sh
# or on Windows
emsdk_env.bat
If yor are using PowerShell on Windows you might need allow script access:Set-ExecutionPolicy Unrestricted
To verify the installation runemcc -v
emcc --version
Now is the time to create first Hello World. As a Hello World we will use our first C Hello World
#include <stdio.h>
int main()
{
printf("Hello, World from C\n");
return 0;
}
Save it as hello_world.cpp and to compile run:
emcc hello_world.cpp -o hello_world.html --emrun
(Note first time we will run the compiler it might take a which as it will download additional components)
-o – output we want: could be *.html, *.js, or *.wasm
–emrun – we want testable code
To quickly test our first applicaion we can use Emscripten test tool:
emrun hello_world.html
If everything went correctly you should see a browser open with out test page:
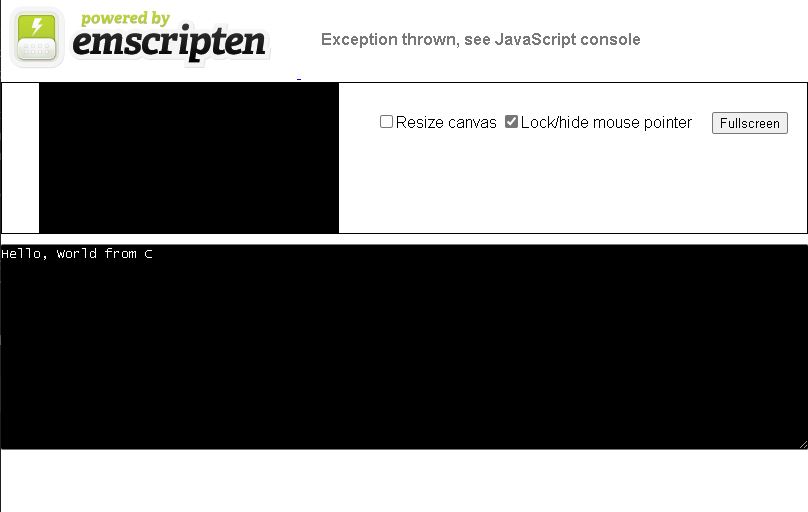
And if you upload all three new files (hello_world.html, hello_world.js, hello_world.wasm) to your web site and point your browser to hello_world.html you should see the same page.
Locally you can also use Python to run web-server: browse to the folder with your page and run:
python -m http.server
If you need just web assembly file use command line parameter -o:
emcc hello_world.cpp -o hello_world.wasm
Emscripten is a wrapper around LLVM compiler, so many it has flags coming from it. For example optimization:
emcc hello_world.cpp -o hello_world.wasm -Oz -std=c++17 //optimized for size
emcc hello_world.cpp -o hello_world.wasm -O3 -std=c++17 //optimized for speed